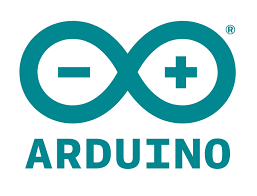
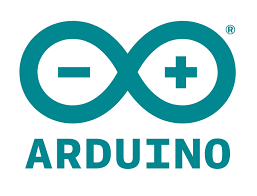
Programme simple pour utiliser un afficheur LCD et utilisation des boutons associés
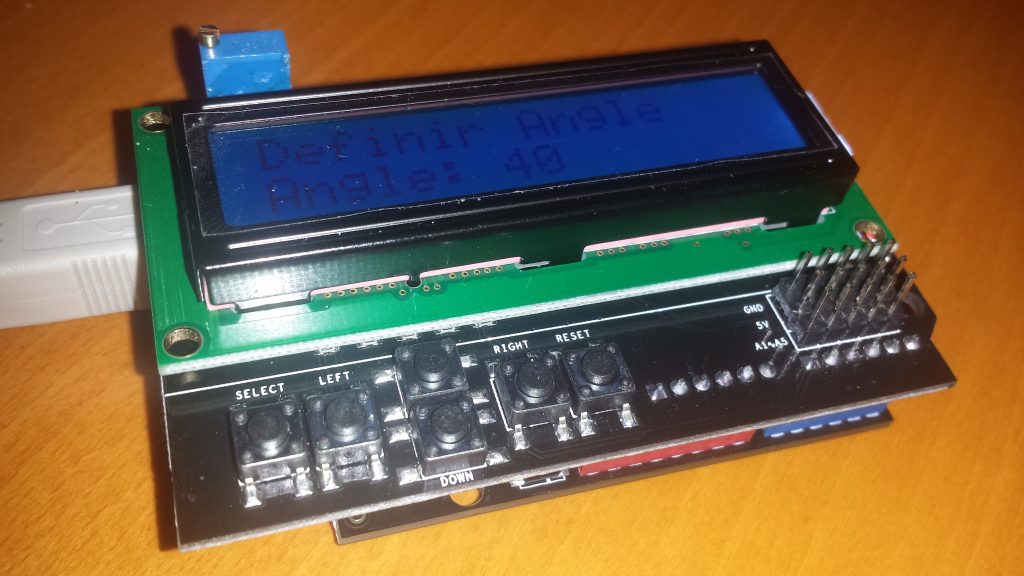
Matériel
- Ecran LCD 16×2 – RB-ITE-161
- Arduino UNO compatible – RB-DFR-189
Code
#include // Include the LCD library #include // Button #define btnRIGHT 1 #define btnUP 2 #define btnDOWN 4 #define btnLEFT 8 #define btnSELECT 16 #define btnNONE 32 // Initialize the library with the numbers of the interface pins LiquidCrystal lcd(8, 9, 4, 5, 6, 7); // Return the first pressed button found byte read_LCD_buttons() { // read the value from the sensor int adc_key_in = analogRead(A0); if (adc_key_in > 1000) return btnNONE; if (adc_key_in < 50) return btnRIGHT; if (adc_key_in < 250) return btnUP; if (adc_key_in < 450) return btnDOWN; if (adc_key_in < 650) return btnLEFT; if (adc_key_in < 850) return btnSELECT; return btnNONE; // when all others fail, return this. } // Return the number of digits in an integer byte countDigits(int num) { byte count=0; while(num) { num = num / 10; count += 1; } return count; } // Display full screen of text (2 rows x 16 characters) void displayScreen(char row1[], char row2[]) { lcd.clear(); lcd.setCursor(0,0); lcd.print(row1); lcd.setCursor(0,1); lcd.print(row2); Serial.println(""); Serial.println(row1); Serial.println(row2); } // Display to wait for select and then do so void waitSelect(bool disp = false) { if(disp) { lcd.setCursor(0,1); lcd.print("< press select >"); Serial.println("< press select >"); } while(read_LCD_buttons() != btnSELECT){}; } // Displays text on screen and an ADC value at chosen position from selected pin. Waits for user input (select button by default) int getValueADC(char row1[], char row2[], byte pos, byte pin, byte endButton) { int value = 0; int lastValue = -1; byte buttons = btnNONE; displayScreen(row1, row2); delay(1500); while(buttons != endButton) { // Check inputs buttons = read_LCD_buttons(); // Update readout from analog port value = analogRead(pin); // Update display if(value != lastValue) { lcd.setCursor(pos, 1); lcd.print(" "); lcd.setCursor(pos, 1); lcd.print(value); Serial.println(""); Serial.println(row1); Serial.print(row2); Serial.print(" "); Serial.println(value); lastValue = value; delay(350); } }; // Return the last measured ADC value return (value); } // Displays text on screen and a changeable value at chosen position. User input can change the value (left/right/up/down) and press select to accept it. int getValueInRange(char row1[], char row2[], byte pos, int valueDefault, int valueMin, int valueMax, int valueStep, int valueStepLarge) { int value = valueDefault; int lastValue = -1; int buttons = btnNONE; displayScreen(row1, row2); delay(1500); while(buttons != btnSELECT) { // Check inputs buttons = read_LCD_buttons(); switch(buttons) { case btnUP: value += valueStep; if(value > valueMax) value = valueMax; break; case btnRIGHT: value += valueStepLarge; if(value > valueMax) value = valueMax; break; case btnDOWN: value -= valueStep; if(value < valueMin) value = valueMin; break; case btnLEFT: value -= valueStepLarge; if(value < valueMin) value = valueMin; break; } // Update display if(value != lastValue) { lcd.setCursor(pos, 1); lcd.print(" "); lcd.setCursor(pos, 1); lcd.print(value); Serial.println(""); Serial.println(row1); Serial.print(row2); Serial.print(" "); Serial.println(value); lastValue = value; delay(350); } }; // Return the last selected value return (value); } // Timing management long display_time_step = 1000; long display_time = 0; int angle = 45; void setup() { // Initialize serial communication Serial.begin(115200); // Initialize LCD screen lcd.begin(16, 2); // Intro screen displayScreen("Val Technologie", "Pipe Bender"); delay(2500); } void loop() { //Demander angle angle = getValueInRange("Definir Angle", "Angle:", 7, angle, 1,180,1,5); // Up/down screen displayScreen("Pipe Bender", "Pliage en cours"); delay(1000); // Display Etiquette Angle lcd.setCursor(0, 1); lcd.print(" "); lcd.setCursor(0, 1); lcd.print("ANGLE:"); // Display Angle for (int x = 0; x < angle; x++) { lcd.setCursor(13, 1); lcd.print(" "); lcd.setCursor(13, 1); lcd.print(x); delay(50); } // Up/down screen displayScreen("Pipe Bender", "Fin du Pliage"); delay(1000); }